[C++类和对象]类和对象的引入
面向过程和面向对象
C语言是面向过程的,关注的是过程,分析出求解问题的步骤,通过函数调用来逐步解决问题
C+++是基于面向对象的,关注的是对象,将一件事情分成不同的对象,靠对象之间完成交互
类的引入
C语言结构体中只能定义变量,在C++中,结构体不仅仅可以定义变量,而且可以定义函数,
例如 :之前用C语言实现的栈,结构体中只能定义变量,现在已C++的方式实现,不仅可以定义变量,而且还可以在结构体定义函数
对于结构体的定义,在C++中 更喜欢用class来定义
class className
{//类体:由成员函数和成员变量来组成
};//后面一定要写分号
class为定义类的关键字,className为类的名字,{}中为类的主体,注意类定义结束之后后面的分号不能省略
类体中内容称为类的成员:类的变量称为类的属性或成员变量;类中的函数被称为类的方法或者是类的成员函数
类的两种第一方式
1.声明和定义全部放在类体中,需要主义:成员函数如果在类中定义,编译器可能会把函数当作内联函数来做处理
2.类声明放在.h文件中,成员函数定义在.cpp的文件中,
如果这样的话,要在函数前加上类名
C++里类域中的成员变量通常书写前加一个_区分,可以写在前面例如:_year,或者year_
例如:我们的成员变量如果使用的year不进行区分
传入的变量year = year month = month day = day,通常会报错
因为编译器无法区分变量是函数形参还是成员变量
class Date
{
public:void Init(int year, int month, int day){_year = year;_month = month;_day = day;}private:int _year; // year_ m_yearint _month;int _day;
};int main()
{Date d;d.Init(2024, 3, 31);return 0;
}
类的访问限定符及封装
访问限定符
c++实现封装的方式:用类将对象的属性与方法结合到一块,让对象更加完善,通过访问权限选择将其接口提供给外部的用户使用
1. public修饰的成员在类外可以直接被访问
2. protected和private修饰的成员在类外不能直接被访问(此处protected和private是类似的)
3. 访问权限作用域从该访问限定符出现的位置开始直到下一个访问限定符出现时为止
4. 如果后面没有访问限定符,作用域就到 } 即类结束。
5. class的默认访问权限为private,struct为public(因为struct要兼容C) 注意:访问限定符只在编译时有用,当数据映射到内存后,没有任何访问限定符上的区别
封装
面向对象的三大特性?
封装 继承 多态
在类和对象阶段,主要是研究类的封装性,什么事封装?
封装:将数据和操作数据的方法进行有机结合,隐藏对象的属性和实现细节,仅对外公开接口来进行和对象进行交互.
封装的本质是一种管理,让用户更方便使用类
在C++中实现封装,可以通过类将数据以及操作数据的方法进行有机结合,通过访问权限来隐藏对象内部实现细节,控制哪些方法可以在类外部直接被使用.
类的作用域
类定义了一个新的作用域,类的所有成员都在类的作用域中.在类体外定义成员时,需要使用::作用域操作符指明成员属于哪个类域
class Person
{
public:void PrintPersonInfo();
private:char _name[20];char _gender[3];int _age;
};
// 这里需要指定PrintPersonInfo是属于Person这个类域
void Person::PrintPersonInfo()
{cout << _name << " "<< _gender << " " << _age << endl;
}
类的实例化
用类类型创建对象的过程,称为类的实例化
1.类是对对象进行描述的,是一个模型一样的东西,限定了类有哪些成员,定义出一个类并没有分配实际的内存空间来存储他.
2.一个类可以实例化出多个对象,实例化出的对象,占用实际的物理空间,存储类成员变量
class Person
{
public:void PrintPersonInfo();
private:char _name[20];char _gender[3];int _age;
};
int main()
{Person._age = 100;
// 编译失败:error C2059: 语法错误:“.”
//并且成员变量都是私有的不能直接使用return 0;
}
Person类是没有空间的,只有Person类实例化的对象才有具体的空间
打个比方,类实例化出对象就像现实中使用建筑设计图建造出房子,类就像是设计图,只设计出需要什么东西,但没有实体存在,同样类也是一个设计,实例化出的对象才能实际存储数据,占用物理空间
类对象模型
如何计算类对象的大小
class A
{
public:void PrintA(){cout<<_a<<endl;}
private:char _a;
};
类中既可以有成员变量,又可以有成员函数,那么一个类的对象中包含了什么?如何计算一个类的大小
类对象的存储方式猜测
对象中包含类的各个成员
// 类中既有成员变量,又有成员函数
class A1 {
public:void f1(){}
private:int _a;
};
// 类中仅有成员函数
class A2 {
public:void f2() {}
};
// 类中什么都没有---空类
class A3
{};
this指针
我们先来定义一个日期类
class Date
{
public:void Init(int year, int month, int day){_year = year;_month = month;_day = day;}//void Print(Date* this)void Print(){cout << _year << "-" << _month << "-" << _day << endl;}
private:int _year; // 年int _month; // 月int _day; // 日int a;
};
int main()
{Date d1, d2;d1.Init(2022, 1, 11);d2.Init(2022, 1, 12);d1.Print();d2.Print();return 0;
}
class Date
{
public:void Init(int year, int month, int day){_year = year;_month = month;_day = day;}//void Print(Date* const this)void Print(){//cout <<this-> _year << "-" << this->_month << "-" <<this-> _day << endl;cout << _year << "-" << _month << "-" << _day << endl;}
private:int _year; // 年int _month; // 月int _day; // 日int a;
};
int main()
{Date d1, d2;d1.Init(2022, 1, 11);d2.Init(2022, 1, 12);//d1.Print(&d1);d1.Print();//d2.Print(&d2);d2.Print();return 0;
}
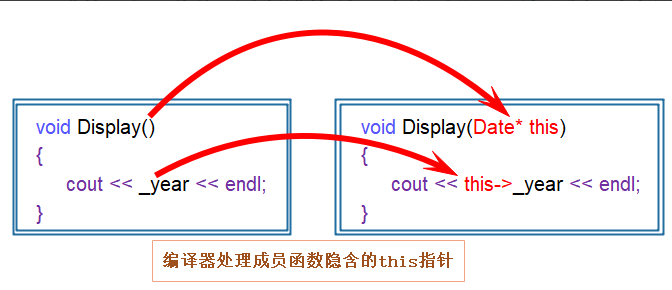
this指针存在哪里?
this指针可以为空吗?
传递空指针不会报编译错误,没有访问,所以正常运行
class A
{
public:void Print(){cout << "Print()" << endl;}
private:int _a;
};
int main()
{A* p = nullptr;p->Print();return 0;
}
// 1.下面程序编译运行结果是? A、编译报错 B、运行崩溃 C、正常运行
运行崩溃
class A
{
public:void PrintA(){cout << _a << endl;//cout << this->_a << endl;}
private:int _a;
};
int main()
{A* p = nullptr;//p->PrintA(&p);p->PrintA();return 0;
}
this
通常不占用对象内存,而是作为隐藏参数传递,可能存栈上。
有些存在ecx寄存器上
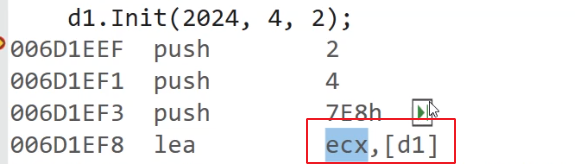
把C语言中的结构体用C++重新实现一下
#include <iostream>
#include <stdlib.h>
#include <stdbool.h>
using namespace std;typedef int DataType;
class Stack
{
public:void Init(int n = 4){_arry = (DataType*)malloc(sizeof(DataType)*n);if (_arry == NULL){perror("malloc is fail");return;}_size = 0;_capacity = n;}int size(){return _size;}bool empty(){return _size == 0;}void push(DataType data){Checkcapacity();_arry[_size] = data;_size++;}void Pop(){if (empty()){return;}_size--;}int top(){return _arry[_size - 1];}void Destory(){free(_arry);_arry = nullptr;_capacity = 0;_size = 0;}
private:void Checkcapacity(){if (_size == _capacity){int newcapacity = _capacity * 2;DataType* temp = (DataType*)realloc(_arry, newcapacity * sizeof(DataType));if (temp == NULL){perror("realloc fail");return;}_arry = temp;_capacity = newcapacity;}}
private:DataType* _arry;int _size;int _capacity;
};int main()
{Stack st;st.Init();st.push(1);st.push(2);st.push(3);st.push(4);st.push(5);while (!st.empty()){ cout << st.top() << " ";st.Pop();}return 0;
}